Python is a versatile and powerful programming language that’s great for beginners due to its simplicity and readability. Whether you want to break into the world of programming, data science, web development, or automation, learning Python is a valuable first step. This guide will walk you through the essential skills you can acquire through various Python online courses designed specifically for beginners.
1. Basic Syntax and Programming Concepts
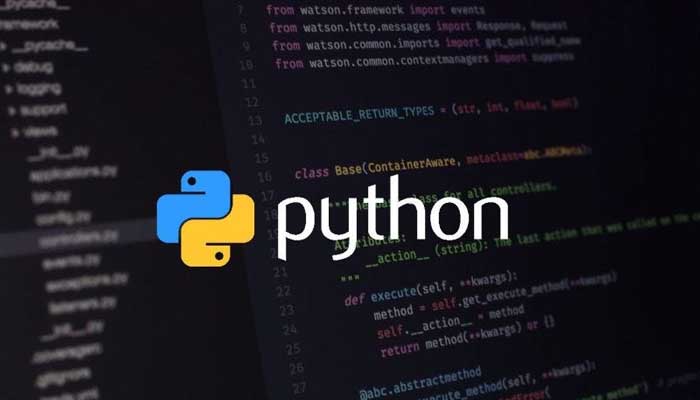
Every beginner course in Python starts with understanding the basic syntax and core programming concepts. These foundational skills include:
- Variables and Data Types: Learn how to declare variables and work with different data types like integers, floats, strings, and booleans.
- Operators: Understand arithmetic, comparison, and logical operators to perform various operations on data.
- Control Flow: Master if-else statements, loops (for and while), and control flow to make decisions and repeat tasks.
- Functions: Learn how to define and call functions, pass parameters, and return values to organize code into reusable blocks.
Recommended Courses:
- Python for Everybody by Coursera
- Complete Python Bootcamp: Go from Zero to Hero in Python 3 by Udemy
2. Data Structures
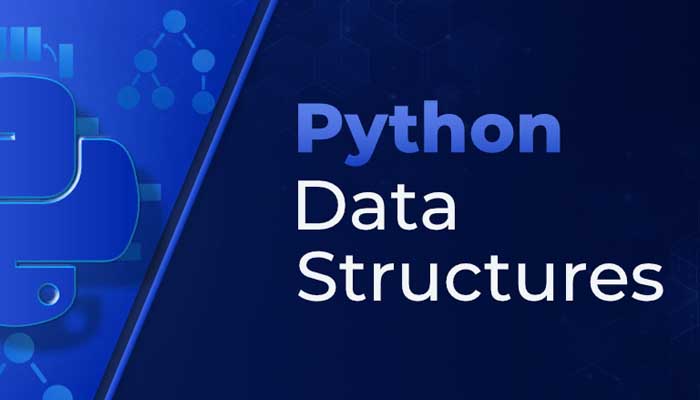
Data structures are crucial for organizing and storing data efficiently. Beginners will learn:
- Lists: Create and manipulate lists to store ordered collections of items.
- Tuples: Understand immutable sequences and their use cases.
- Dictionaries: Use key-value pairs to store data and perform lookups.
- Sets: Work with unordered collections of unique items.
Recommended Courses:
- Learn Python Programming Masterclass by Udemy
- Python Basics by Codecademy
3. Input and Output
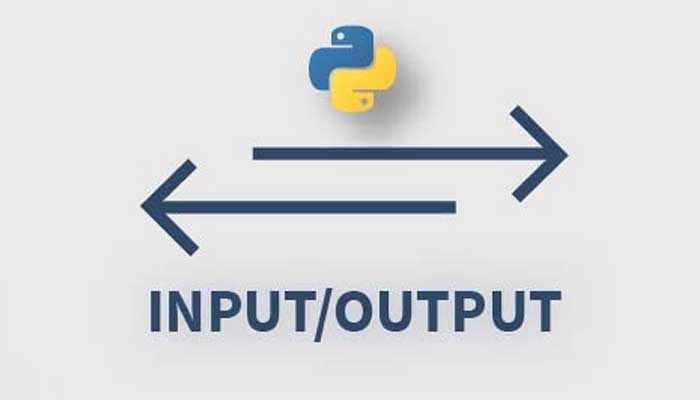
Handling user input and producing output are fundamental skills:
- Reading User Input: Learn to use the input() function to get data from users.
- File I/O: Understand how to read from and write to files, handle file paths, and manage file modes (read, write, append).
Recommended Courses:
- Automate the Boring Stuff with Python by Udemy
- CS50’s Introduction to Computer Science by edX
4. Error Handling
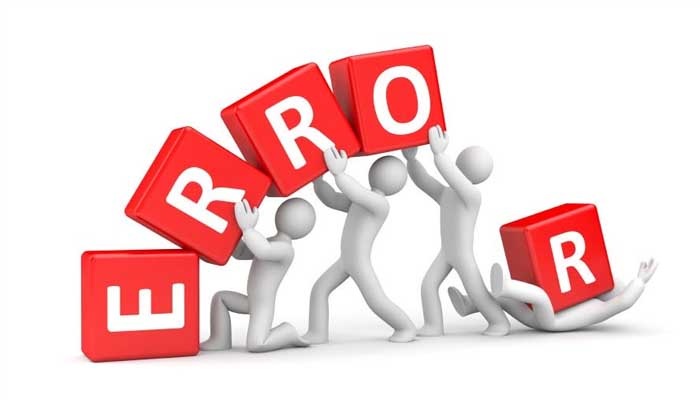
Writing robust programs requires effective error handling:
- Exceptions: Learn about try-except blocks to catch and handle errors gracefully.
- Custom Exceptions: Understand how to create and raise custom exceptions for specific error conditions.
Recommended Courses:
- Introduction to Python Programming by Udacity
- Google IT Automation with Python Professional Certificate by Coursera
5. Modules and Packages
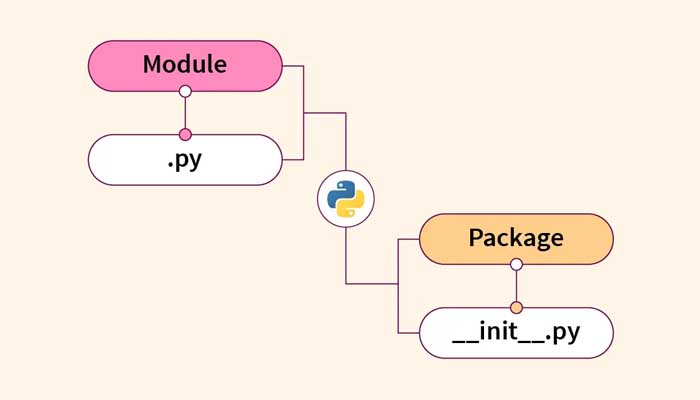
Modules and packages help in organizing and reusing code:
- Importing Modules: Learn how to import standard and third-party modules.
- Creating Modules: Understand how to write your own modules and packages.
- Using Libraries: Get introduced to popular Python libraries like NumPy, Pandas, and Matplotlib.
Recommended Courses:
- Python 3 Programming Specialization by Coursera
- The Python Mega Course: Build 10 Real World Applications by Udemy
6. Object-Oriented Programming (OOP)
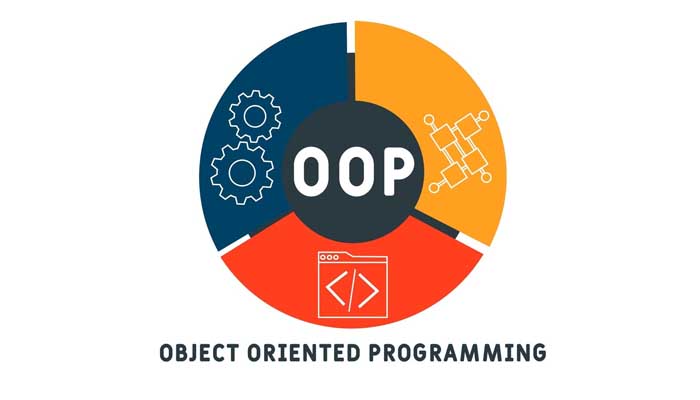
Object-oriented programming is a powerful paradigm in Python:
- Classes and Objects: Learn how to define classes and create objects.
- Inheritance: Understand how to inherit properties and methods from other classes.
- Encapsulation and Polymorphism: Master these OOP principles to write more modular and maintainable code.
Recommended Courses:
- Complete Python Developer in 2024: Zero to Mastery by Udemy
- Introduction to Computer Science and Programming Using Python by edX
7. Web Scraping
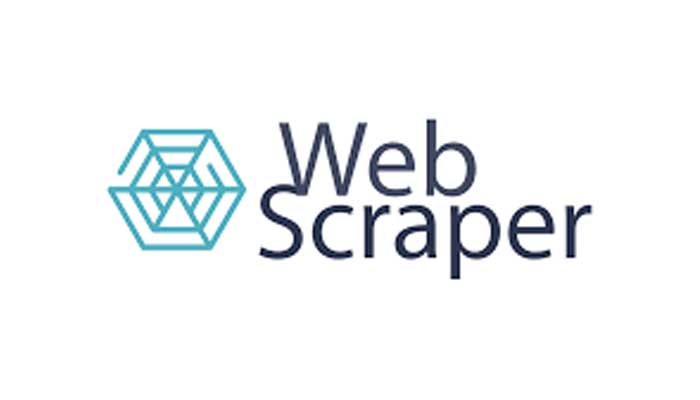
Web scraping allows you to collect data from websites:
- BeautifulSoup: Learn to parse HTML and XML documents to extract data.
- Requests: Understand how to send HTTP requests to access web content.
- Automation with Selenium: Get introduced to web automation using the Selenium library.
Recommended Courses:
- Python and Web Scraping by Codecademy
- Web Scraping with Python by Udemy
8. Working with APIs
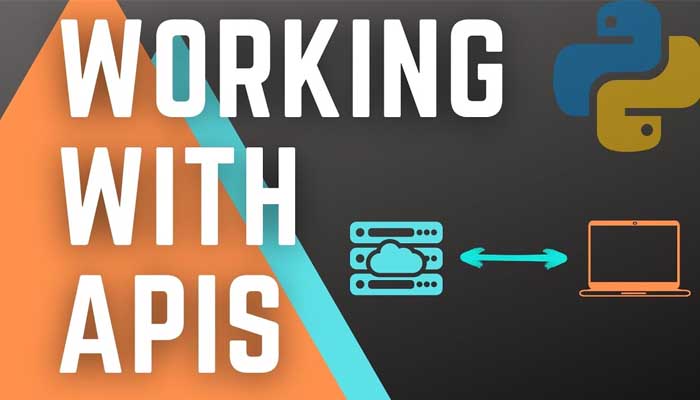
APIs (Application Programming Interfaces) enable interaction with external services:
- Making API Calls: Learn how to use the requests library to make GET and POST requests.
- Handling JSON Data: Understand how to parse and manipulate JSON data returned from APIs.
Recommended Courses:
- Python for Data Science and Machine Learning Bootcamp by Udemy
- APIs and Web Scraping with Python by DataCamp
9. Introduction to Data Science
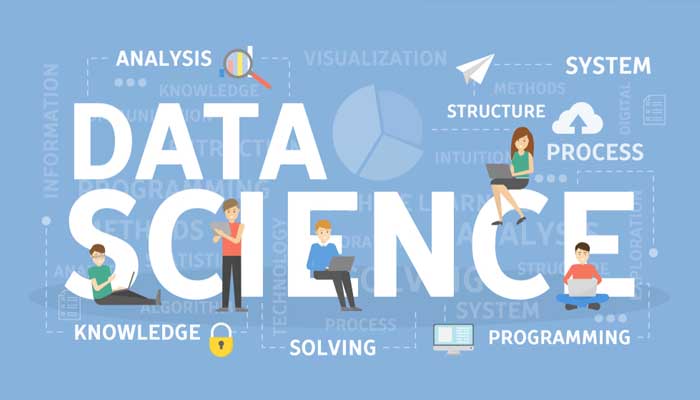
Python is widely used in data science:
- NumPy: Learn how to perform numerical operations on large datasets.
- Pandas: Understand how to manipulate and analyze data using DataFrames.
- Matplotlib and Seaborn: Get introduced to data visualization techniques.
Recommended Courses:
- Python for Data Science by Coursera
- Data Science with Python by edX
10. Version Control with Git
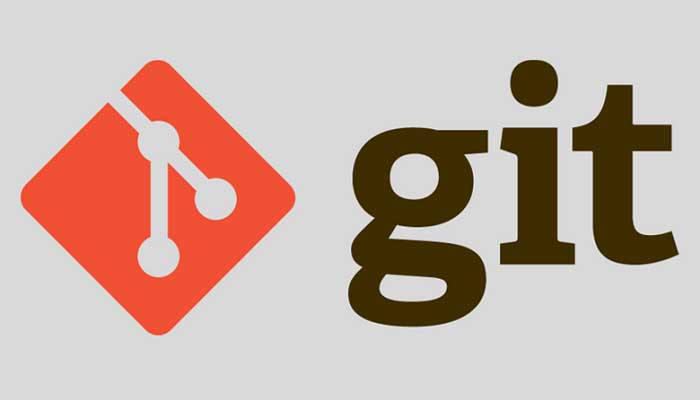
Version control is essential for collaborative programming:
- Git Basics: Learn how to initialize a repository, commit changes, and track versions.
- GitHub: Understand how to push code to GitHub, manage repositories, and collaborate with others.
Recommended Courses:
- Google IT Automation with Python Professional Certificate by Coursera
- Version Control with Git by Coursera
11. Building Simple Web Applications
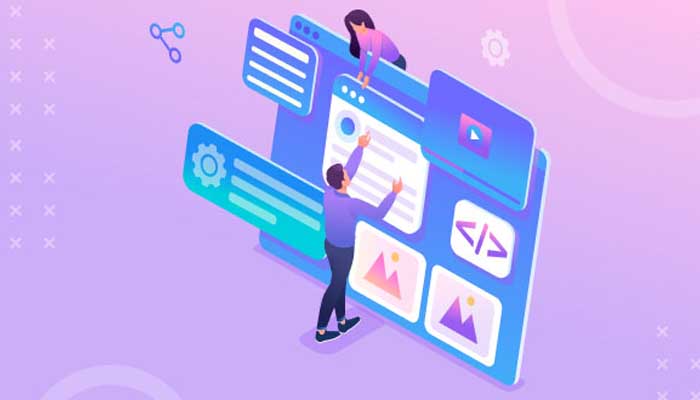
Creating web applications is a practical application of Python skills:
- Flask: Get introduced to the Flask web framework to build simple web applications.
- Django: Understand the basics of the Django framework for more robust web development.
Recommended Courses:
- Python and Django Full Stack Web Developer Bootcamp by Udemy
- Web Application Technologies and Django by Coursera
12. Practice Projects and Capstone Projects
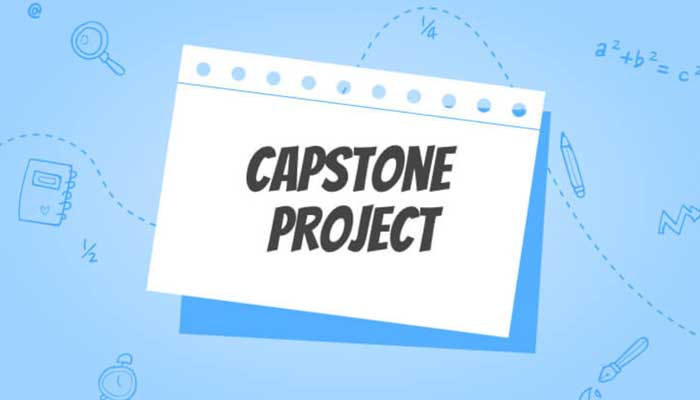
Applying what you’ve learned through projects is crucial:
- Small Projects: Work on small projects like calculators, to-do lists, and simple games.
- Capstone Projects: Engage in larger projects that integrate multiple skills, such as developing a web app or a data analysis pipeline.
Recommended Courses:
- Complete Python Bootcamp: Go from Zero to Hero in Python 3 by Udemy
- Google IT Automation with Python Professional Certificate by Coursera
Conclusion
Learning Python opens up a world of opportunities in various fields like web development, data science, automation, and more. The essential skills outlined in this guide provide a comprehensive roadmap for beginners. By taking the recommended online courses, you can build a solid foundation in Python programming, equipping yourself with the knowledge and practical experience needed to advance in your career. Invest in your learning journey today and harness the power of Python to transform your professional life in 2024.